Machine learning ஐ படிக்க தொடங்குவதற்கு முதலில் நாம் Jupyter notebook ஐ தெரிந்துக் கொள்ள வேண்டும். நீங்கள் இதை Arvin Education YouTube Channel வாயிலாகவும் தெரிந்து கொள்ளலாம்.
Jupyter notebook என்றால் என்ன ?
Jupyter notebook என்பது ஒரு Open source web application ஆகும். இது code, narrations மற்றும் equations எழுதுவதற்கும் மற்றும் visualizations செய்வதற்கும் பயன்படுகிறது.
இப்பொழுது Jupyter notebook ஐ எப்படி Start செய்வது என்பதை பார்க்கலாம்.
முதலில் Start button ஐ அழுத்தி Anaconda3 (64-bit) > Anaconda Navigator (Anaconda3) என்ற Option ஐ கீழே உள்ள Screenshot போல Select செய்யுங்கள்.

Anaconda Navigator Open ஆனவுடன் அதில் கீழே உள்ள Screenshot போல Launch button ஐ Click செய்யுங்கள். இப்பொழுது Jupyter notebook start ஆகி விடும்.
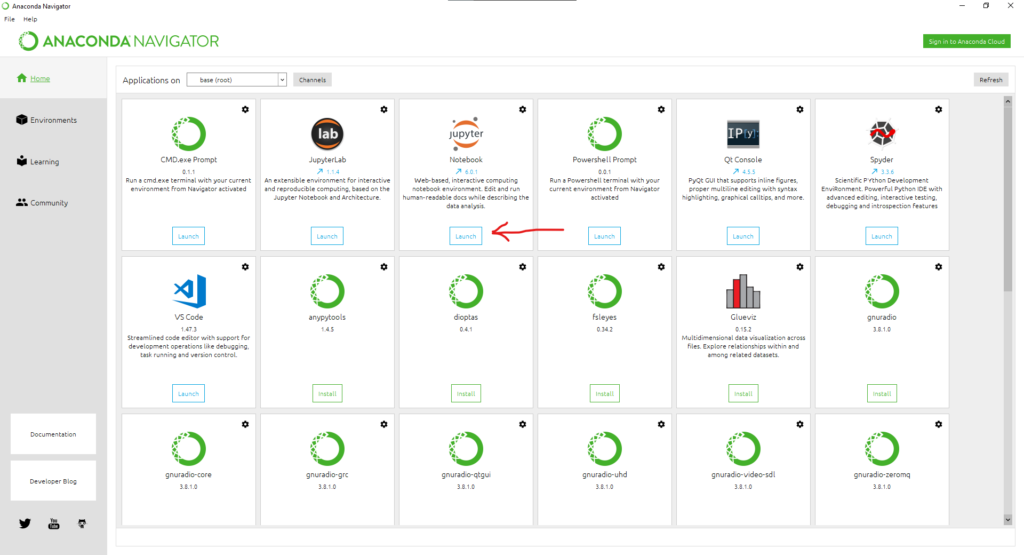
Jupyter notebook start ஆனவுடன் கீழே உள்ள Screenshot போல இருக்கும்.

இது Jupyter notebook இன் File explorer section.இதில் உங்களுக்கு பிடித்த Folder ஐ Select செய்து கொள்ளவும்.அதன் பிறகு New button ஐ Click செய்து python 3 என்ற option ஐ Select செய்யவும்.
இப்பொழுது ஒரு புது Notebook உருவாகிவிடும். அது கீழே உள்ள Screenshot போல இருக்கும்.
மேலே உள்ள screenshot இல் Jupyter interface பற்றி தெளிவாக ஆங்கிலத்தில் குறிப்பிடப்பட்டுள்ளது. இதில் உள்ள Shortcut களும் ஆங்கிலத்தில் குறிப்பிடப்பட்டுள்ளது.
நாம் இப்பொழுது ஒரு Basic Python program ஐ பார்ப்போம். கீழே உள்ள Code ஐ Copy செய்து Cell இல் Paste செய்யுங்கள்.
print('hello world')
நமக்கு கீழே உள்ள Screenshot போல output கிடைக்கும்.
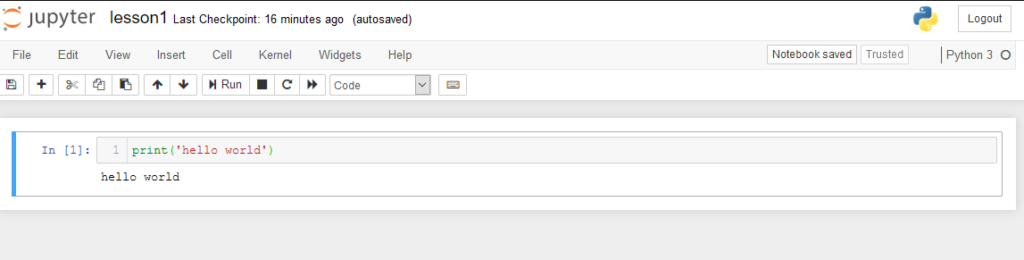
சரி நண்பர்களே அடுத்த post இல் variables, functions, machine learning specific packages numPy, Pandas, Sklearn, Tensorflow, Kares, பற்றி பார்போம்.
நீங்கள் இந்த post ஐ ஆங்கிலத்தில் படிக்க விரும்பினால் கீழே உள்ள Link ஐ Click செய்யவும்.
நன்றி நண்பர்களே
இந்த Post இல் பிழை இருந்தால் மன்னிக்கவும்.